【Python】ttk:tkinterと比べた各ウィジェットの外観と機能面の違い
2021.01.06 /
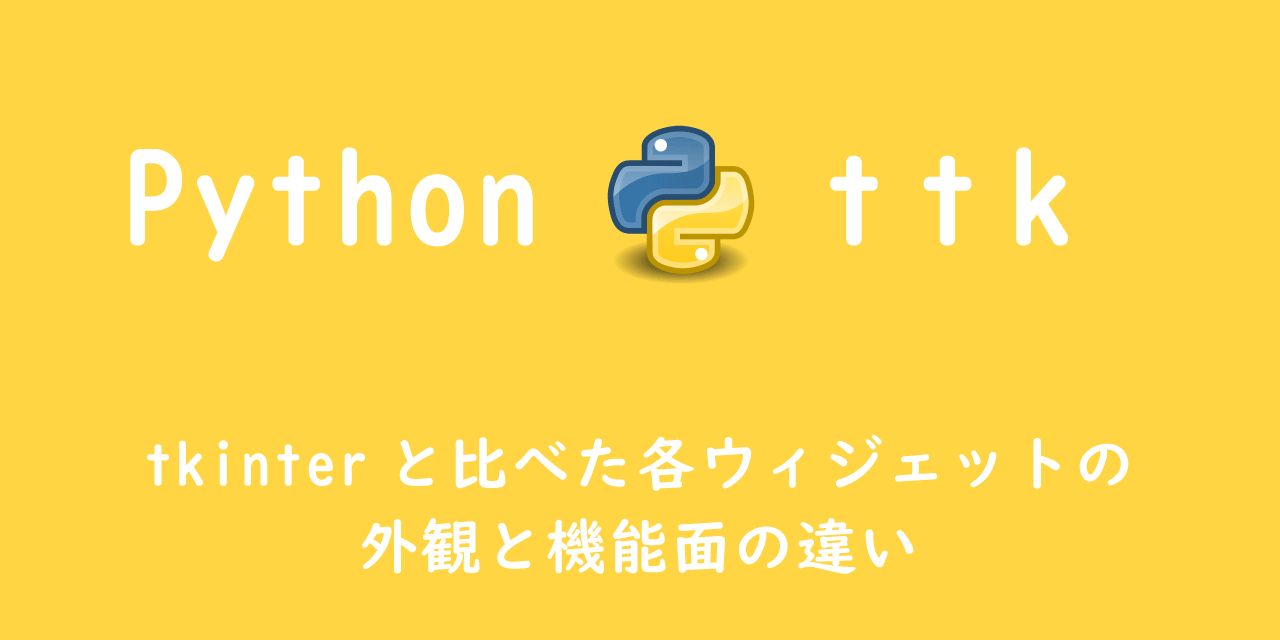
モダンなGUIアプリケーションをPythonで作成できるtkinter.ttkが通常のtkinterと比べてどのように外観や機能面の違いについてまとめました。
よりオシャレでモダンなGUIアプリを作成するならttkを使うのがお勧めです。
商用向けにGUIアプリの開発を進めている方や、社内向けアプリの場合でもユーザーインターフェースを良くしたい方は必ずチェックしておいてください。
本記事を通して以下の知識を学べます。
- PythonでモダンなGUIアプリの作成方法
- ウィジェットの種類や使い方
- tkinterとttkの外観や機能面の違い
tkinter、ttkとは
tkinter
tkinterとはPythonによるGUIアプリケーションを作成するモジュールの一つです。
標準ライブラリなので別でインストールする必要はないです。
tkinterを使ったGUIアプリはクロスプラットフォームなので、OSを気にすることなく作成したアプリを使用できます。
基本的なtkinterの使い方は以下記事をご参照ください。
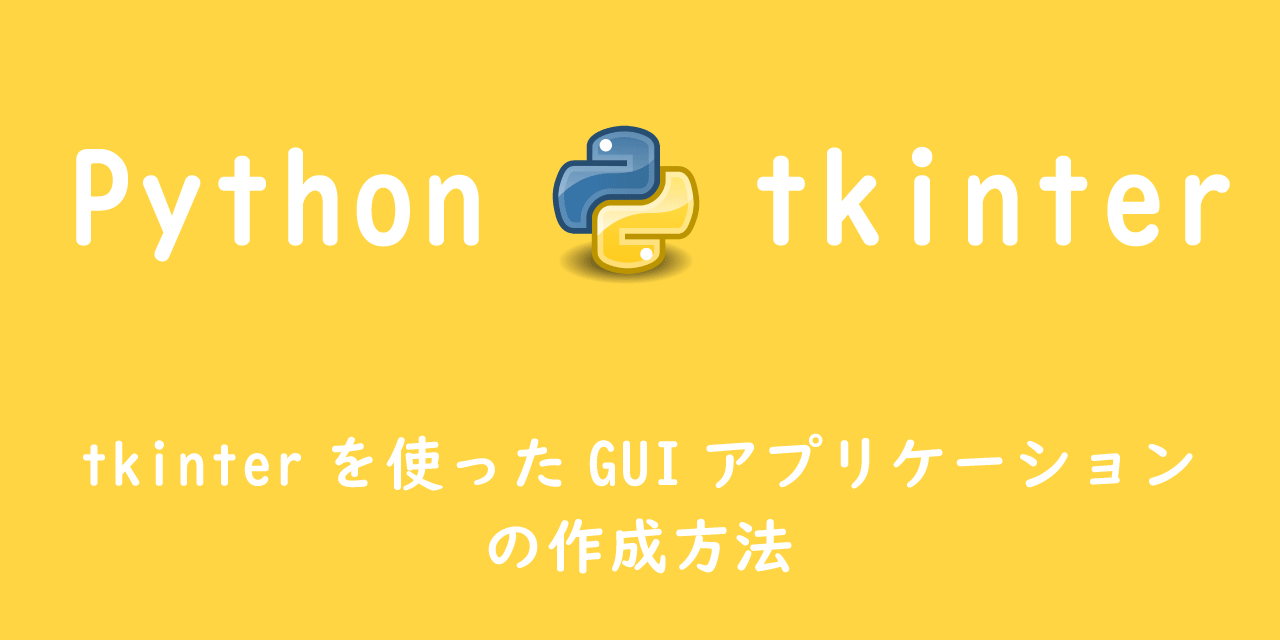
【Python】tkinterを使ったGUIアプリケーションの作成方法
ttk
tkinter.ttkはTk 8.5から導入されました。
ttkは通常のtkinterに比べて各ウィジェットの外観がモダンになり、機能も充実しました。
ttkを使うためにはtkinterからttkをインポートする必要があります。
from tkinter import ttk
ttkにはtkinterに含まれる12種類のウィジェットと新たに6種類のウィジェットを加えて計18種類のウィジェットが存在します。
- Button(tkinterに含まれる)
- Checkbutton(tkinterに含まれる)
- Entry(tkinterに含まれる)
- Frame(tkinterに含まれる)
- Label(tkinterに含まれる)
- LabelFrame(tkinterに含まれる)
- Menubutton(tkinterに含まれる)
- PanedWindow(tkinterに含まれる)
- Radiobutton(tkinterに含まれる)
- Scale(tkinterに含まれる)
- Scrollbar(tkinterに含まれる)
- Spinbox(tkinterに含まれる)
- Combobox(ttkのみ)
- Notebook(ttkのみ)
- Progressbar(ttkのみ)
- Separator(ttkのみ)
- Sizegrip(ttkのみ)
- Treeview(ttkのみ)
ttkを使うことで外観が良くなるだけでなく、ユーザーの使いやすさが向上します。
tkinterと比べて書式の変更方法やオプション設定の仕様が異なるので注意してください。
tkinterとttkの各ウィジェットの外観の違い
Button
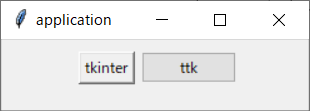
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
button = tk.Button(frame, text="tkinter")
button_ttk = ttk.Button(frame, text="ttk")
# 各種ウィジェットの設置
button.grid(row=0, column=0, padx=5)
button_ttk.grid(row=0, column=1)
root.mainloop()
ttkのボタンウィジェットはtkinterと比べて外眼は大きく変化しています。
よりモダンになっているのは一目でわかるかと思います。
機能面ですと、マウスカーソルがボタンに重なると色が変わるようになっています。
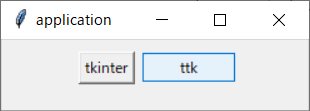
ユーザーの使いやすさは非常に良くなっていることがわかります。
ボタンウィジェットの詳しい機能面については以下記事をご参照ください。
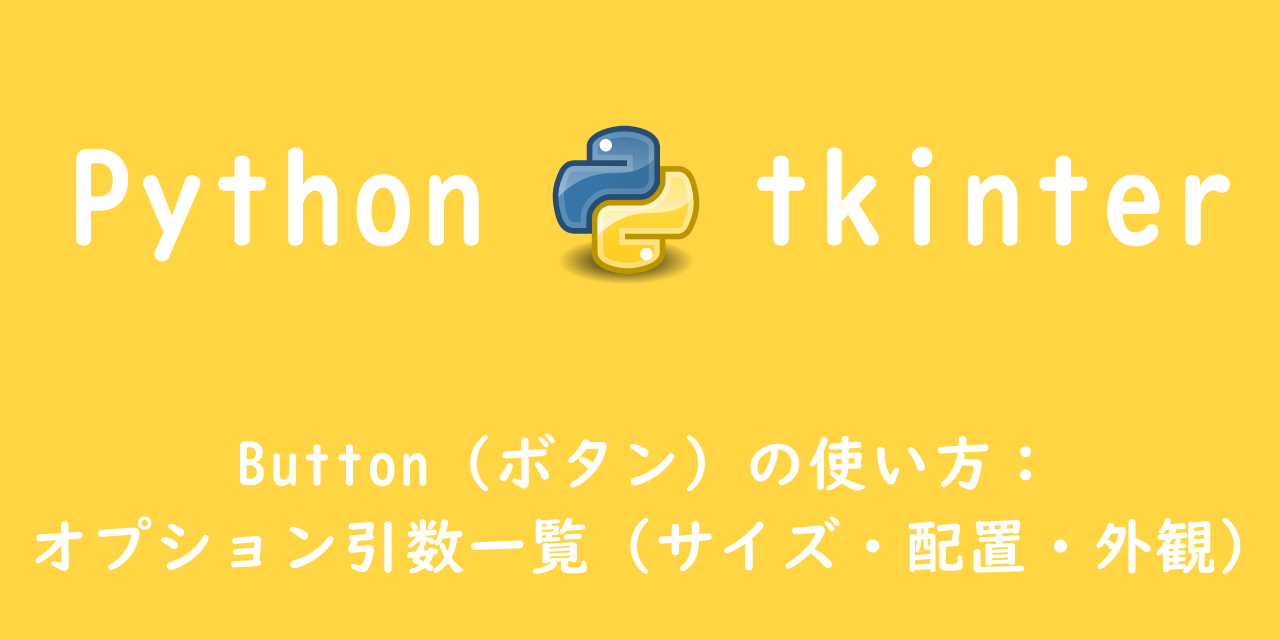
【Python Tkinter】Button(ボタン)の使い方:オプション引数一覧(サイズ・配置・外観)
Checkbutton
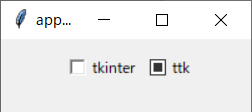
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
checkbutton = tk.Checkbutton(frame, text="tkinter")
checkbutton_ttk = ttk.Checkbutton(frame, text="ttk")
# 各種ウィジェットの設置
checkbutton.grid(row=0, column=0, padx=5)
checkbutton_ttk.grid(row=0, column=1)
root.mainloop()
Entry
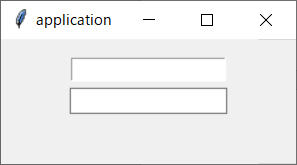
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
entry = tk.Entry(frame, text="tkinter")
entry_ttk = ttk.Entry(frame, text="ttk")
# 各種ウィジェットの設置
entry.grid(row=0, column=0, pady=5)
entry_ttk.grid(row=1, column=0)
root.mainloop()
外観は大きくは変化はありませんが機能面で大きな違いがあります。
マウスカーソルをEntryウィジェットに重ねるとttkの場合、枠線の色が太くなり強調されます。
またEntryウィジェットで文字入力が可能状態にした際に、ttkでは枠線の色が変化します。
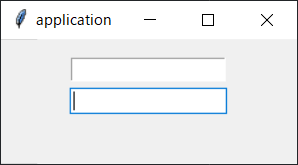
Label
ラベルはtkinterとttkでは大きな違いは見られません。
ラベルの詳しい使い方については以下記事をご参照ください。
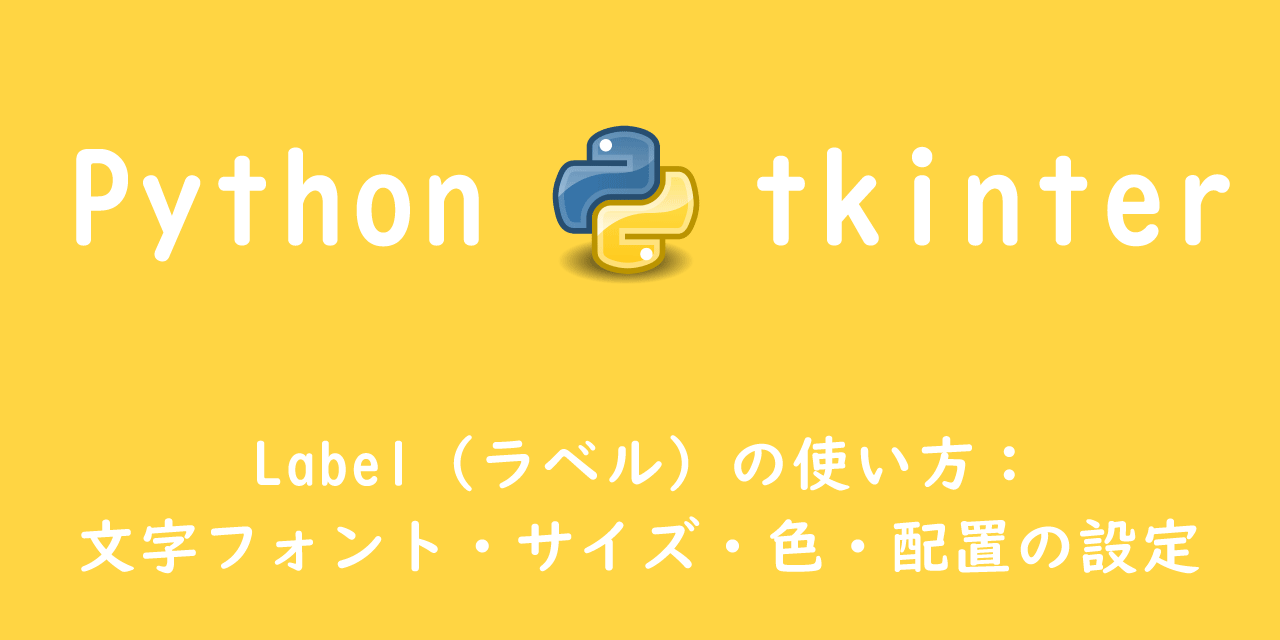
【Python/Tkinter】Label(ラベル)の使い方:文字フォント・サイズ・色・配置の設定
Menubutton
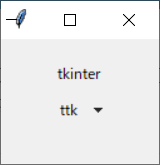
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
menubutton = tk.Menubutton(frame, text="tkinter")
menubutton_ttk = ttk.Menubutton(frame, text="ttk")
# 各種ウィジェットの設置
menubutton.grid(row=0, column=0, pady=5)
menubutton_ttk.grid(row=1, column=0)
root.mainloop()
Radiobutton
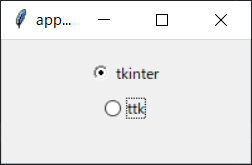
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
radio = tk.Radiobutton(frame, text="tkinter")
radio_ttk = ttk.Radiobutton(frame, text="ttk")
# 各種ウィジェットの設置
radio.grid(row=0, column=0, pady=5)
radio_ttk.grid(row=1, column=0)
root.mainloop()
見た目に大きな違いはありませんが、機能面で違いがあります。
ttkのラジオボタンはウィジェットにカーソルを載せるとラジオボタンの枠が変化します。
Scale
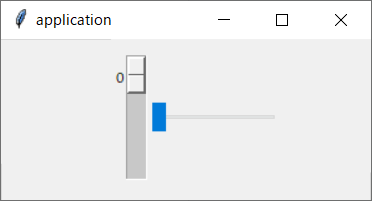
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
scale = tk.Scale(frame)
scale_ttk = ttk.Scale(frame)
# 各種ウィジェットの設置
scale.grid(row=0, column=0)
scale_ttk.grid(row=0, column=1)
root.mainloop()
Scaleウィジェットとは、つまみを左右に動かすことしパラメータ・設定を感覚的に変更できるウィジェットです。
Spinbox
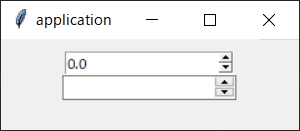
import tkinter as tk
from tkinter import ttk
# rootメインウィンドウの設定
root = tk.Tk()
root.title("application")
root.geometry("200x100")
# メインフレームの作成と設置
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
# 各種ウィジェットの作成
radio = tk.Spinbox(frame, format='%3.1f', increment=0.1, from_=0, to=5)
radio_ttk = ttk.Spinbox(frame, format='%3.1f', increment=0.1, from_=0, to=5)
# 各種ウィジェットの設置
radio.grid(row=0, column=0)
radio_ttk.grid(row=1, column=0)
root.mainloop()
Spinboxウィジェットとは、入力欄(Entryウィジェット)の横に数値を増減できるスピンボタンが付いているウィジェットです。
tkinterとttkでは外観に若干の違いがあります。
機能面では、ttkではマウスカーソルがウィジェット上に重なると枠線が強調される違いがあります。